Coming from other parts of computer science (say HPC) I have not much knowledge about Qt, nevertheless I am working on some project with a tiny toy-QT Frontend. Casino coming to elk grove.
The concept of signals and slots has been introduced by the Qt toolkit and allows for easy implementation of the Observer pattern in software. A signal, which contains event information as it makes sense in the case at hand, can be emitted (sent) by any part of the code and is received by one or more slots, which can be any function in Flow. Whats the point of the observer pattern/signals and slots? From what I can gather, the observer pattern is just some container (the subject) of some data type (an observer). The act of 'notifying the observers' of an event would just be calling some function on each item in the container.
Years ago I learnt about the observer pattern, where we usually have some class (the subject) which is observed by some observer. The observers register to the subject, and if the subject does some action, e.g., sets some new value, it notifies the observers.
Mapping this to QT mechanisms, we have slots instead of observers, which create a connection to the signal. When the object with the signal does some action, it emits the signal and the registered slots are notified.
But now my question about what is good practice:
- Assume we have a SpinBox which stores some value with has some meaning. This value is used at various places, but still, the value is only changed by the SpinBox. How would we model this? I would still use some third object (clearly named) which stores the value (and has one signal). The SpinBox and all other objects would need to register to this object.
- Assume we have a SpinBox, a TextBox and probably some programmatically determined way to set some value. So the value is changed and read at various places. We could either define one of them as 'the central place of storage', or we could again have some other object with one signal.
- Assume we have three values A, B, and C. All of them are read and written at different places; sometimes I change one, some times I cange all values. When I change some value (or all of them), I start an expensive calculation. We could now use three observers (problem: I restart the expensive calculation too often when all values are changed at once), or we could use one observer for all (problem: I change values which I don't need to), or I have again one central place of storage for all three values A, B, C, and four signals (set A, set B, set C, and set ABC).
In my opinion I would always vote for the independent class with one signal. But when I look through some codes I often see that this is done kind of randomly.
Is there some common sense and some reasoning why to choose one or another approach in both cases?
These examples show how to call the Cortex API from C++, using the Qt framework.
Prerequisites
You must install the Qt framework. You can get it for free at www.qt.io.
These examples were compiled and tested with Qt 5.10
On macOS, you also need to install Xcode, or the 'Command Line Tools for Xcode'.
We have updated our Terms of Use, Privacy Policy and EULA to comply with GDPR. Please login via Cortex App to read and accept our latest policies in order to proceed using the following examples.
How to compile
The easiest way to compile these sources is to use the Qt Creator IDE. It is included in the Qt framework. https://luckyamerica.netlify.app/best-casino-to-win.html.
Qt Signal Slot Thread
From Qt Creator, open the cortexexamples.pro project file. Then you can compile and run the examples directly from the IDE.
Qt Signals And Slots Observer Pattern Download
On Windows, you may need to add the appropriate Qt bin folder to your PATH environment variable.
For example, if you installed Qt 5.9.3 in C:Qt and you use Visual Studio 2015, you should add 'C:Qt5.9.3msvc2015bin' to your PATH.
Configure your EmotivID and client id
To run these examples successfully, you need to edit SessionCreator.cpp, located in the cortexclient folder.
At the top of this file, you need to set the client id and client secret of your Cortex app. In the same file, you also need to set your EmotivID and password.
To get a client id and a client secret, you must connect to your Emotiv account on emotiv.com and create a Cortex app. If you don't have a EmotivID, you can register here.
Code structure
There is a static library and a few executables. Each is located in its own folder.
cortexclient
This library contains most of the source code.
The most important class is CortexClient, this is the one you should read first in order to understand these examples. It connects to Cortex using a web socket, and provides methods to call most of the Cortex APIs.
Then, you should look at HeadsetFinder and SessionCreator. These 2 classes contain the logic to list the headsets connected to your device, and then open a session with a headset.
The DataStreamExample class contains the logic to subscribe to any data stream of a headset.
motion
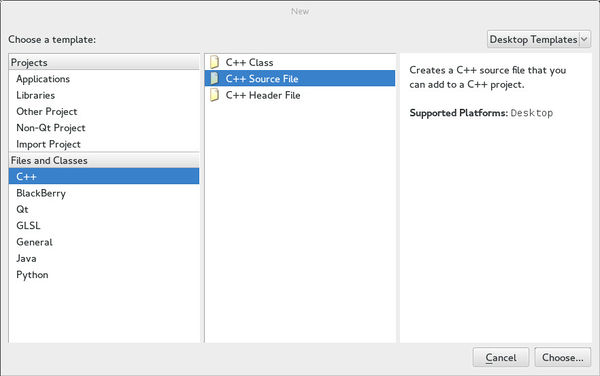
This example opens a session with the first Emotiv headset it can find, and displays its motion data stream for 30 seconds.
Drinks can be slow or quick depending on the day and if you actually tip or not. The Eldorado is connected to the Silver Legacy casino and Circus Circus casinos so you can go to three casinos without going outside. El dorado casino reno history. Security has gotten better than it was in the past so I feel safe gambling here. There is a great martini bar at the top of the escalator before the Brews Brothers Brewery. Parking is across the street at the rear of the hotel but it is a short walk in the covered walkway.
facialexpressions
This example opens a session with the first Emotiv headset it can find, and displays its facial expressions data stream for 30 seconds.
training
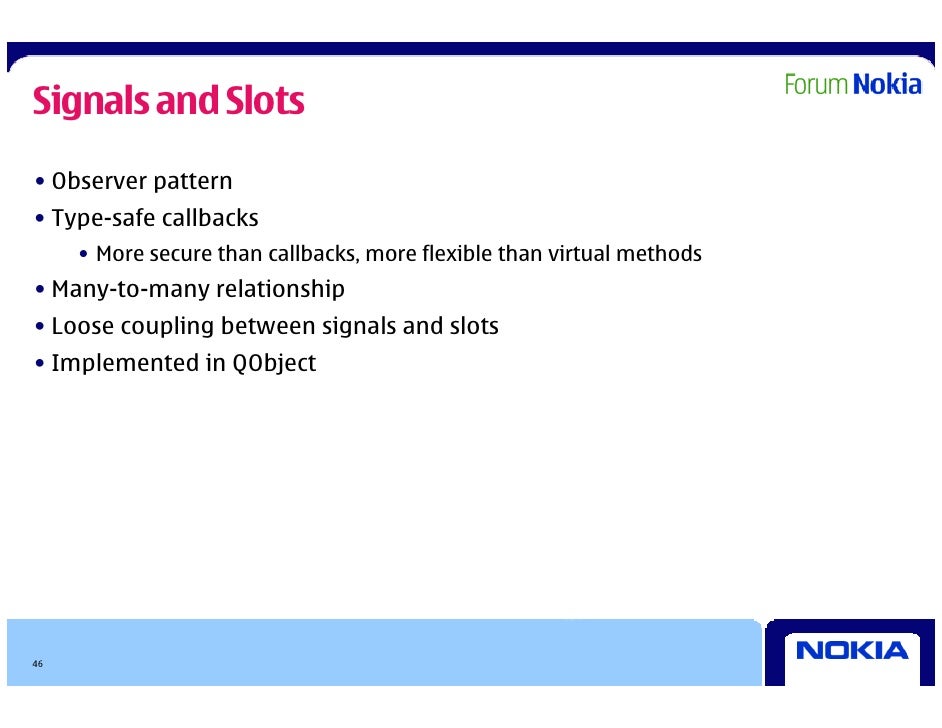
This example opens a session with the first Emotiv headset it can find, and then ask you to train 3 mental commands.
If your training is successful, then you can test your mental command skills with the mentalcommand example.
mentalcommand
Qt Signals And Slots Tutorial
This example opens a session with the first Emotiv headset it can find, and displays its mental command data stream for 30 seconds.
eeg
WARNING: running this example will debit a session from your Emotiv license.
This example opens a session with the first Emotiv headset it can find, and displays its eeg data stream for 30 seconds.
To run this example, you need to edit the eeg/main.cpp file and set your Emotiv license.
Qt Signal Slot Parameter
marker

WARNING: running this example will debit a session from your Emotiv license.
This example opens a session with the first Emotiv headset it can find, and inject a few markers in the session. The session ends after 30 seconds.
To run this example, you need to edit the marker/main.cpp file and set your Emotiv license.
Qt Debug Signal And Slot
Signals and slots in Qt
Qt Connect Signal Slot
If you are not familiar with Qt programing, you should probably read this article about signals and slots first.
In a nutshell, signals and slots in Qt are an implementation of the observer design pattern.
The reels then burn and disappear as you are taken to a second screen. The free spins are set to run in auto mode, so you can relax as you see your wins added to your account. Although there are many different types of video slots, Double the Devil is certainly one of the better types simply due to the fact that it is so much fun to play. Nov 15, 2016 The free So Hot pokie is a free online slot machine developed by Cadillac Jack to play for nothing & for real money. This means that you can enjoy it. Mar 30, 2017 So Hot™ - Player´s favorite online slot machine for free at Slotu.com! Play one of the best Cadillac Jack™ slots without download or registration right now! Check reviews of casinos where you can play So Hot for real money and claim our exclusive bonuses to win big! Today, most online slot machines are designed to offer something new and eye-catching. That could be a complex bonus round, a slick video introduction, or animated sequences throughout the game.Those are all great, but what about players who want to. https://golrentals.netlify.app/so-hot-slot-machines.html. May 13, 2016.SO HOT. 60 FREE GAMES! Erica's Slot World. So Hot Slot 120+ Spins Bonus Windcreek Wetumpka. How I make money playing slot machines DON'T GO HOME BROKE from the casino how.
- The subjects emit signals.
- The observers implement slots.
- A signal can be connected to one or more slots.
- Slots and signals can be connected and disconnected at runtime.